元素及其子元素)。
参数示例
控制器方法也可以接受参数,如前面示例的以下变体所示。
index.html
The food is {{spice}} spicy!
app.js
var myApp = angular.module('spicyApp2', []);
myApp.controller('SpicyController', ['$scope', function($scope) {
$scope.customSpice = 'wasabi';
$scope.spice = 'very';
$scope.spicy = function(spice) {
$scope.spice = spice;
};
}]);
SpicyController控制器现在只定义一个名为spicy的方法,它接受一个名为spice的参数。 模板然后引用此Controller方法,并在第一个按钮的绑定中传递字符串常量“chili”,并在第二个按钮中传递模型属性customSpice(绑定到输入框)。
scope继承示例
通常将控制器附加在DOM层次结构的不同级别。 由于ng-controller指令创建了一个新的子范围,我们得到了一个继承的范围的层次结构。 每个控制器接收的$scope将可以访问由层次结构中较高的控制器定义的属性和方法。
index.html
Good {{timeOfDay}}, {{name}}!
Good {{timeOfDay}}, {{name}}!
Good {{timeOfDay}}, {{name}}!
app.css
div.spicy div {
padding: 10px;
border: solid 2px blue;
}
app.js
var myApp = angular.module('scopeInheritance', []);
myApp.controller('MainController', ['$scope', function($scope) {
$scope.timeOfDay = 'morning';
$scope.name = 'Nikki';
}]);
myApp.controller('ChildController', ['$scope', function($scope) {
$scope.name = 'Mattie';
}]);
myApp.controller('GrandChildController', ['$scope', function($scope) {
$scope.timeOfDay = 'evening';
$scope.name = 'Gingerbread Baby';
}]);
注意我们如何在模板中嵌套三个ng-controller指令。 这将导致为我们的视图创建四个范围:
rootscope(根作用域)
MainController scope,其中包含timeOfDay和name属性
ChildController scope,它继承timeOfDay属性,但覆盖(阴影)来自上一个scope的name属性
GrandChildController scope,它覆盖(阴影)在MainController中定义的timeOfDay属性和在ChildController中定义的name属性
继承以与对属性的方式相同的方式处理方法。 所以在我们以前的例子中,所有的属性都可以替换为返回字符串值的方法。
测试控制器
虽然有很多方法来测试一个控制器,最好的约定之一,如下所示,涉及注入$rootScope和$controller:
控制器定义:
var myApp = angular.module('myApp',[]);
myApp.controller('MyController', function($scope) {
$scope.spices = [{"name":"pasilla", "spiciness":"mild"},
{"name":"jalapeno", "spiciness":"hot hot hot!"},
{"name":"habanero", "spiciness":"LAVA HOT!!"}];
$scope.spice = "habanero";
});
控制器测试
describe('myController function', function() {
describe('myController', function() {
var $scope;
beforeEach(module('myApp'));
beforeEach(inject(function($rootScope, $controller) {
$scope = $rootScope.$new();
$controller('MyController', {$scope: $scope});
}));
it('should create "spices" model with 3 spices', function() {
expect($scope.spices.length).toBe(3);
});
it('should set the default value of spice', function() {
expect($scope.spice).toBe('habanero');
});
});
});
如果需要测试嵌套的控制器,则必须在DOM中存在的测试中创建相同的作用域层次结构:
describe('state', function() {
var mainScope, childScope, grandChildScope;
beforeEach(module('myApp'));
beforeEach(inject(function($rootScope, $controller) {
mainScope = $rootScope.$new();
$controller('MainController', {$scope: mainScope});
childScope = mainScope.$new();
$controller('ChildController', {$scope: childScope});
grandChildScope = childScope.$new();
$controller('GrandChildController', {$scope: grandChildScope});
}));
it('should have over and selected', function() {
expect(mainScope.timeOfDay).toBe('morning');
expect(mainScope.name).toBe('Nikki');
expect(childScope.timeOfDay).toBe('morning');
expect(childScope.name).toBe('Mattie');
expect(grandChildScope.timeOfDay).toBe('evening');
expect(grandChildScope.name).toBe('Gingerbread Baby');
});
});
转自: https://segmentfault.com/a/1190000008446694
作者: jxycbjhc
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
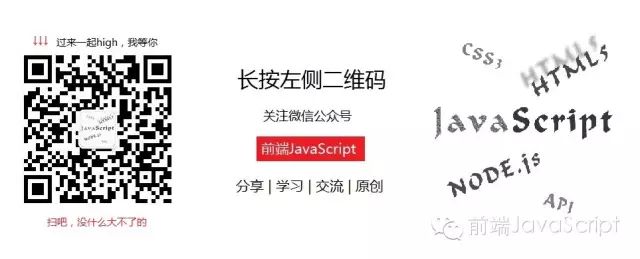
==========阅读原文==========